Python语法
使用Python 3 语法
运行环境:jupyter notebook#变量:变量赋值末尾不用加;
a=2
b=3
#变量的运算
c=a+b
#使用print打印变量
print(c)
#自由得对变量进行运算
print(8 + c)
#一个*表示乘,两个**表示指数
c=2
print(c**2)
输出:4
#type函数可以显示出值的类型,例如int、string、float、boolean、函数、类等对象
type(100/2)
输出:float
#字符串中单引号和双引号无所谓,还有三个引号,这个就不用回车符
s='''test
test
test'''
print(s
输出:
test
test
test
#字符串与数字的运算
string = 3 * " Ha"
print(string)
输出:Ha Ha Ha
#对字符串进行增加,将字符串进行int类型转换
s = str(1000)+"10"
some_var = "10"
b = 11 + int(some_var)
print(s)
print(b)
输出:
100010
21
#对字符串进行操作,获取字符串第23个字符
letter = "abcdefghijklmnopqrstuvwxyz"
print(letter[22])
print(letter[-2]) #倒数第二个
print(letter[5:10]) #第6-11个
print(letter[::-1]) #[start:end:step]
#字符串切分
string2 = "I like,apple,banana"
str3 = string2.split(',')
print(str3[2])
#字符串替换:
letter.replace('c','a')
#切分用
string2 = "I like,apple,banana"
str3 = string2.split(',')
print(str3[2])
#替换:
letter.replace('c','a')
###使用弹出对话框输入并且显示出来 ###
age = input("How old are you: ")
height = input("how tall(M) are you: ")
weight = input("how much do you weight(kg): ")
print("%s years old,%sM miter height,%skg weight" % (age,height,weight))
#两个数字相加
first = input("first:")
second = input("second:")
print(first,"+",second,"=",int(first)+int(second))
#统计字母的数量
dna = 'ACGTTTCAGGTCA'
dna_cont = {b:dna.count(b)for b in set(dna)}
dna_cont
输出:
{'C': 3, 'G': 3, 'A': 3, 'T': 4}
#这里测试input,并且计算判断过来多少多少天、小时、分钟,同时使用if判断语句
total_minutes = int(input("过了多少分钟:"))
days = total_minutes // 1440
if days != 0:
hours = (total_minutes - days * 1440) // 60
minute = (total_minutes - days * 1440) - hours * 60
else:
hours = total_minutes // 60
minute = total_minutes - hours * 60
print("现在是:",days,"days:",hours,"hours:",minute,"mins")
输出:
过了多少分钟:600【输入值】
现在是: 0 days: 10 hours: 0 mins
#print打印的方式
print('{},{}'.format(1,2))
print("%d, %d" % (1,2))
#数组的表示式:
week = ['mon','tues','wednes','thur']
#打印数组第一个
week[0]
#追加数组
week.append('fri')
print(week)
输出:['mon', 'tues', 'wednes', 'thur', 'fri']
#用sort,type类型必须是一样的
num = [1,4,5,3,2,6]
num.sort()
print(num)
输出:[1, 2, 3, 4, 5, 6]
#Tuple元组,字符串是一个元组,不允许更改里面的值
week_tuple = ('mon','tues','wed')
#week_tuple[0] = fri 不支持
week_tuple
#字典dictionary,
#emty_dict = {} 用大括号 value可以是任何值
emty_dict = {
'one':1,
'two':2,
'three':3,
'four':4,
}
print(emty_dict['two'])
输出:
2
#创建一个字典并且赋值
pizza = {}
pizza['one'] = '1'
pizza['two'] = '2'
print(pizza)
输出:
{'one': '1', 'two': '2'}
#集,像dictionary,但只有key,不能重复的元素,区别list,set,也是用大括号
#空set用:
even_set = set()
even_set = {1,2,2,2,3,4,5,5,5,6,6,7}
even_set2 = {1,3,8,9,10}
even_set
输出:{1, 2, 3, 4, 5, 6, 7}
#Operations not or and
even_set & even_set2 #两个集显示相同的key
even_set | even_set2
#while循环:
week_while = ['mon','tues','wednes','thur']
i = 0
while i < len(week_while):
print(week_while[i])
i += 1
输出:
mon
tues
wednes
thur
#for循环
for i in range(len(week_while)): #i在这里被赋值
print(week_while[i])
obj = ['string',1,True,3.1423]
for ob in obj:
print(ob)
#另一种使用方式
num_list = [i for i in range(2,10)]
num_list
输出:[2, 3, 4, 5, 6, 7, 8, 9]
#嵌套for和if判断语句
num_list = [i for i in range(2,10) if i % 2 == 1]
num_list
#if elif else用法:
rain = 0.7
if rain > 0.6:
print('带雨伞') #缩进判断打印多少
elif rain > 0.6:
print('不带雨伞')
else:
print('不管了')
#while loop一直循环,输入y一直执行下去,直到输入n
cnt = 1
while True:
print('cnt = %d' % cnt)
ch = input("do you want to continue?[y:n]:")
if ch == 'y':
cnt += 1
else:
break
#函数function格式:
def do_noting():
pass
#函数参数中*,表示很多个参数
def print_args(*args):
print('args:',args)
print_args(1,2,3,44,'hello')
输出:
args: (1, 2, 3, 44, 'hello')
#两个星号是字典
def print_keargs(**kwargs):
print('keyword args: ',kwargs)
print_keargs(first = 1,second = 2)
输出:
keyword args: {'first': 1, 'second': 2}
#函数返回值
def other_opt(a,b,c):
return a * b * c
#lambda用法:
double = lambda x:x*2
double(2)
输出:4
#相当于def double(a)
#利用lambda实现需求计算
mang_function = [
(lambda x:x**2),
(lambda x:x**3),
(lambda x:x**4)
]
print(mang_function[0](2))
print(mang_function[1](2))
print(mang_function[2](2))
输出:
4
8
16
#利用python特殊格式实现功能的简便化
mylist = [1,2,3,4,5,6,7,8,9,12,13,16,18]
[i for i in mylist if i % 2 == 0]
#把函数做成数列,调用数列使其运行
def multiply(x):
return x*x
def add(x):
return x+x
func = [add,multiply]
for i in range(5):
value = list(map(lambda x:x(i),func))
print(value)
#做图函数 matplotlib.pyplot
import matplotlib.pyplot as plt #做图函数
fig, ax01 = plt.subplots()
bal_list=[10500.0, 11025.0, 11576.25, 12155.06, 12762.82, 13400.96, 14071.0, 14774.55, 15513.28, 16288.95, 17103.39, 17958.56, 18856.49, 19799.32, 20789.28, 21828.75, 22920.18, 24066.19, 25269.5, 26532.98]
ax01.plot([i for i in range(1,21)],bal_list)
ax01.set(xlabel='n th year', ylabel='Money', title='How much I got')
ax01.grid()
plt.show()
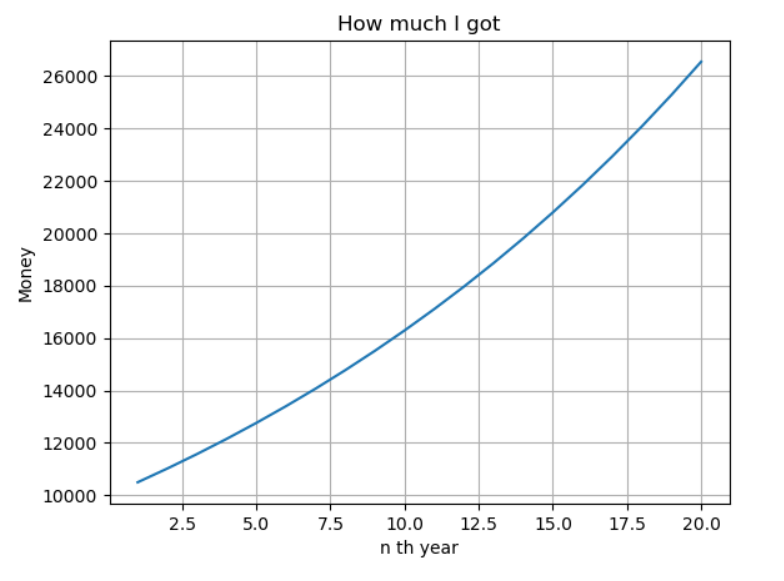
#算法基础,一个算法评价:时间、空间(使用资源)
日期:
from django.utils import timezone
获取今年日期:end_date = timezone.localtime().strftime('%Y%m%d') 格式:20230101
获取昨天的语法:
import datetime
now = datetime.datetime.now()
Yesterdays = (now - datetime.timedelta(hours=23, minutes=59, seconds=59)).strftime('%Y%m%d')